Learning Spring Boot, a robust Java framework, typically requires a dedicated timeframe of 3 to 6 months. At LEARNS.EDU.VN, we help you understand the Spring Boot timeline, the key resources, and industry applications to help you become skilled in this valuable framework. With a structured learning approach, continuous practice, and the right tools, mastering Spring Boot can be achievable within a reasonable timeframe. Explore LEARNS.EDU.VN for comprehensive courses and expert guidance on your path to becoming a proficient Spring Boot developer.
1. Factors Influencing the Learning Timeline: How Long Does It Take To Learn Spring Boot
Several factors influence How Long It Takes To Learn Spring Boot. These include your prior experience with Java, the complexity of the projects you undertake, and your overall dedication. Understanding these factors can help you estimate your personal learning timeline.
1.1. Prior Knowledge of Java
A solid foundation in Java is essential for learning Spring Boot efficiently. Knowledge of core Java concepts, such as object-oriented programming, data structures, and design patterns, can significantly reduce the learning curve. If you’re already familiar with Java, you can focus on learning Spring Boot-specific features and functionalities.
1.2. Project Complexity
The complexity of your Spring Boot projects will directly affect how long it takes to learn the framework. Starting with simple projects, such as building a basic RESTful API, will allow you to grasp the fundamentals before moving on to more complex enterprise applications. As you progress, you’ll encounter new challenges that require deeper understanding and problem-solving skills.
1.3. Dedication and Consistency
Consistent practice and dedication are key to mastering Spring Boot. Allocating time for regular coding, working on projects, and studying documentation will accelerate your learning. The more time and effort you invest, the faster you’ll grasp the core concepts and become proficient in Spring Boot development.
1.4. Learning Resources
Access to high-quality learning resources, such as tutorials, online courses, and books, can significantly impact your learning timeline. These resources provide structured guidance, practical examples, and best practices to help you understand and apply Spring Boot effectively.
1.5. Experience with Related Technologies
Familiarity with related technologies, such as databases, web servers, and build tools, can also influence your learning speed. Spring Boot often integrates with these technologies, so having prior knowledge can make it easier to understand how they work together.
2. A Step-by-Step Guide to Learning Spring Boot
To effectively learn Spring Boot, it’s helpful to follow a structured approach. This step-by-step guide breaks down the learning process into manageable phases, allowing you to build a solid foundation and progress at your own pace. LEARNS.EDU.VN offers comprehensive courses that align with this guide, providing expert guidance and hands-on practice to accelerate your learning journey.
2.1. Setting Up Your Development Environment
Before diving into Spring Boot development, you need to set up your development environment. This includes installing the Java Development Kit (JDK), a Java Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse, and the Spring Boot CLI.
- Install the Java Development Kit (JDK): Download and install the latest version of the JDK from the Oracle website or an open-source distribution like OpenJDK. Ensure that the
JAVA_HOME
environment variable is set correctly. - Install a Java IDE: Choose a Java IDE that suits your preferences. IntelliJ IDEA and Eclipse are popular options, offering features like code completion, debugging, and project management.
- Install the Spring Boot CLI: The Spring Boot CLI simplifies the process of creating and running Spring Boot applications. Install it using a package manager like SDKMAN! or Homebrew, or download the standalone distribution.
2.2. Creating Your First Spring Boot Application
Once your development environment is set up, you can create your first Spring Boot application. This involves creating a new project, adding the necessary dependencies, and writing your first Spring Boot application class.
- Create a New Spring Boot Project: Use the Spring Initializr website (https://start.spring.io/) to generate a new Spring Boot project. Select the desired dependencies, such as
spring-boot-starter-web
for building web applications, and download the generated project. - Open the Project in Your Java IDE: Import the generated project into your Java IDE. This will allow you to edit the code, run the application, and debug any issues.
- Add Dependencies to
pom.xml
: Thepom.xml
file manages the project’s dependencies. Add the necessary dependencies for your application, such as database drivers, security libraries, and testing frameworks. - Write Your First Spring Boot Application Class: Create a Java class annotated with
@SpringBootApplication
. This annotation enables Spring Boot’s auto-configuration and component scanning features. Add amain
method to start the application.
2.3. Implementing Basic Spring Boot Features
With your simple Spring Boot application created, you can start implementing basic Spring Boot features. This includes using annotations, creating controllers, and handling HTTP requests.
- Use Annotations: Spring Boot uses annotations extensively to configure and manage components. Familiarize yourself with common annotations like
@RestController
,@RequestMapping
,@GetMapping
,@PostMapping
, and@Autowired
. - Create Controllers: Controllers handle incoming HTTP requests and return responses. Create controller classes annotated with
@RestController
and define methods to handle specific request mappings using annotations like@GetMapping
and@PostMapping
. - Handle HTTP Requests: Use Spring Boot’s built-in HTTP support to handle HTTP requests and return responses. Extract request parameters, validate input data, and generate appropriate responses in JSON or XML format.
2.4. Working with Databases
Most Spring Boot applications interact with databases to store and retrieve data. Learn how to configure database connections, map Java objects to database tables, and perform CRUD (Create, Read, Update, Delete) operations using Spring Data JPA.
- Configure Database Connections: Configure database connections using Spring Boot’s
application.properties
orapplication.yml
file. Specify the database URL, username, password, and driver class. - Map Java Objects to Database Tables: Use JPA annotations like
@Entity
,@Table
,@Id
,@GeneratedValue
, and@Column
to map Java objects to database tables. Define relationships between entities using annotations like@OneToMany
,@ManyToOne
, and@ManyToMany
. - Perform CRUD Operations: Use Spring Data JPA’s repository interfaces to perform CRUD operations on database tables. Define custom queries using JPQL or native SQL.
2.5. Securing Your Spring Boot Application
Security is a crucial aspect of any web application. Learn how to implement authentication and authorization using Spring Security. Protect your application from unauthorized access, secure sensitive data, and implement role-based access control.
- Add Spring Security Dependency: Add the
spring-boot-starter-security
dependency to your project’spom.xml
file. - Configure Authentication: Configure authentication using Spring Security’s authentication providers. Implement user details services to retrieve user credentials from a database or other source.
- Configure Authorization: Configure authorization using Spring Security’s authorization rules. Define access control rules based on user roles or permissions.
3. Essential Resources and Tools for Learning Spring Boot
To effectively learn Spring Boot, it’s essential to have access to the right resources and tools. Here’s a comprehensive list of books, tutorials, online courses, and community forums that can support your learning journey. LEARNS.EDU.VN also provides curated resources and personalized learning paths to help you master Spring Boot efficiently.
3.1. Books
Books provide a structured and in-depth approach to learning Spring Boot. Here are a few highly recommended options:
Book Title | Author(s) | Description |
---|---|---|
Spring Boot in Action | Craig Walls | A practical guide that covers the fundamentals of Spring Boot, including dependency management, testing, and deployment. |
Spring Boot: Up and Running | Mark Heckler | A beginner-friendly introduction to Spring Boot, covering essential concepts and best practices. |
Spring Boot for Enterprise Applications | Venkat Subramaniam | A comprehensive resource for building enterprise-grade Spring Boot applications, covering topics such as security, performance tuning, and cloud deployment. |
Mastering Spring Boot 2.0 | Dinesh Rajput | This book delves deep into the features of Spring Boot 2.0, offering practical examples and use cases for building microservices and enterprise applications. |
Cloud Native Spring in Action | Josh Long | Focuses on building cloud-native applications with Spring Boot, covering topics like microservices, containerization, and cloud deployment strategies. |
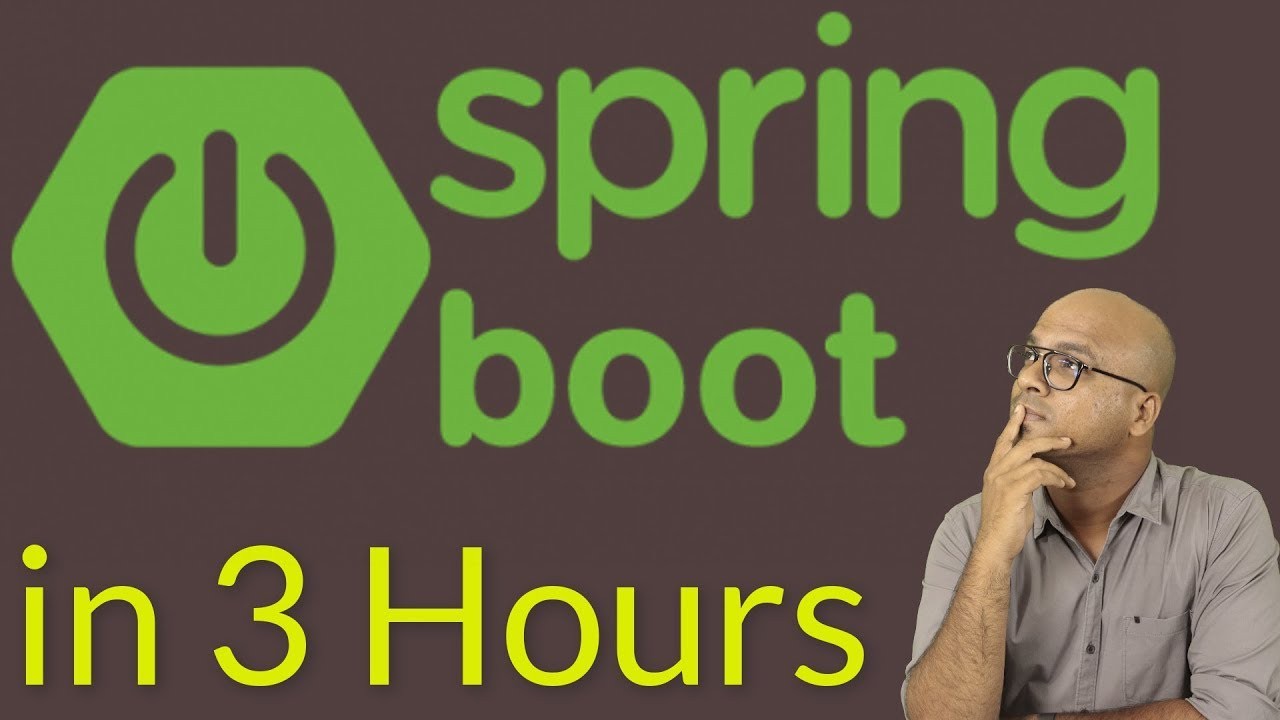
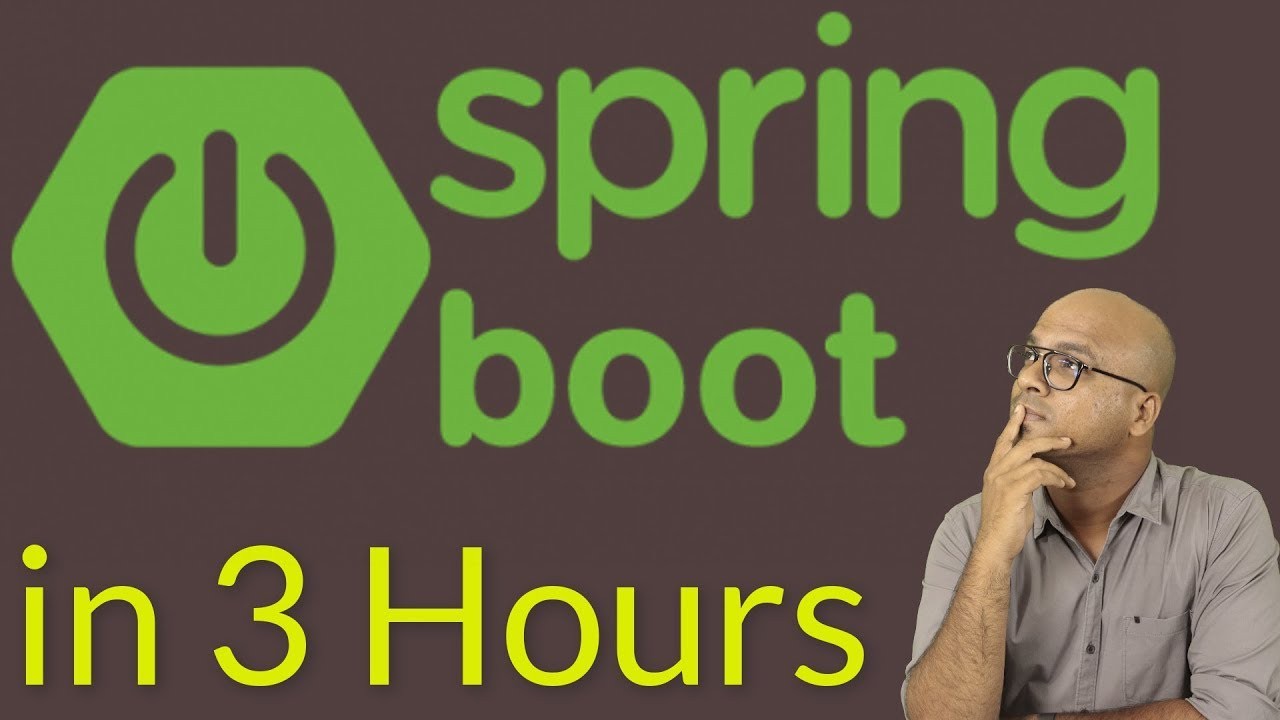
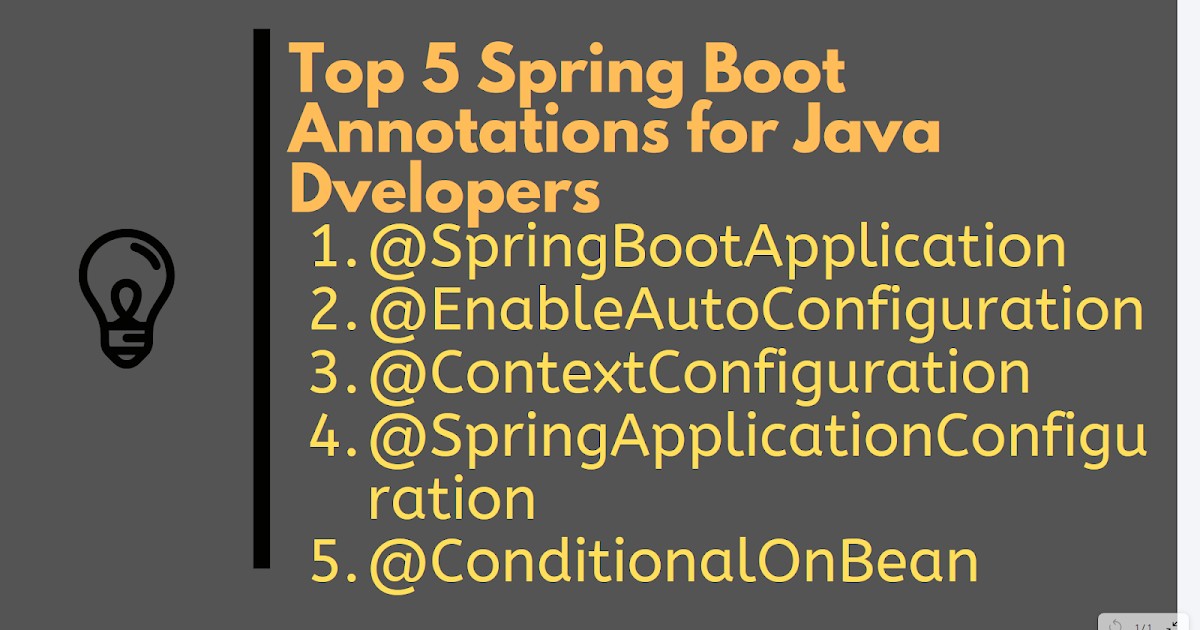
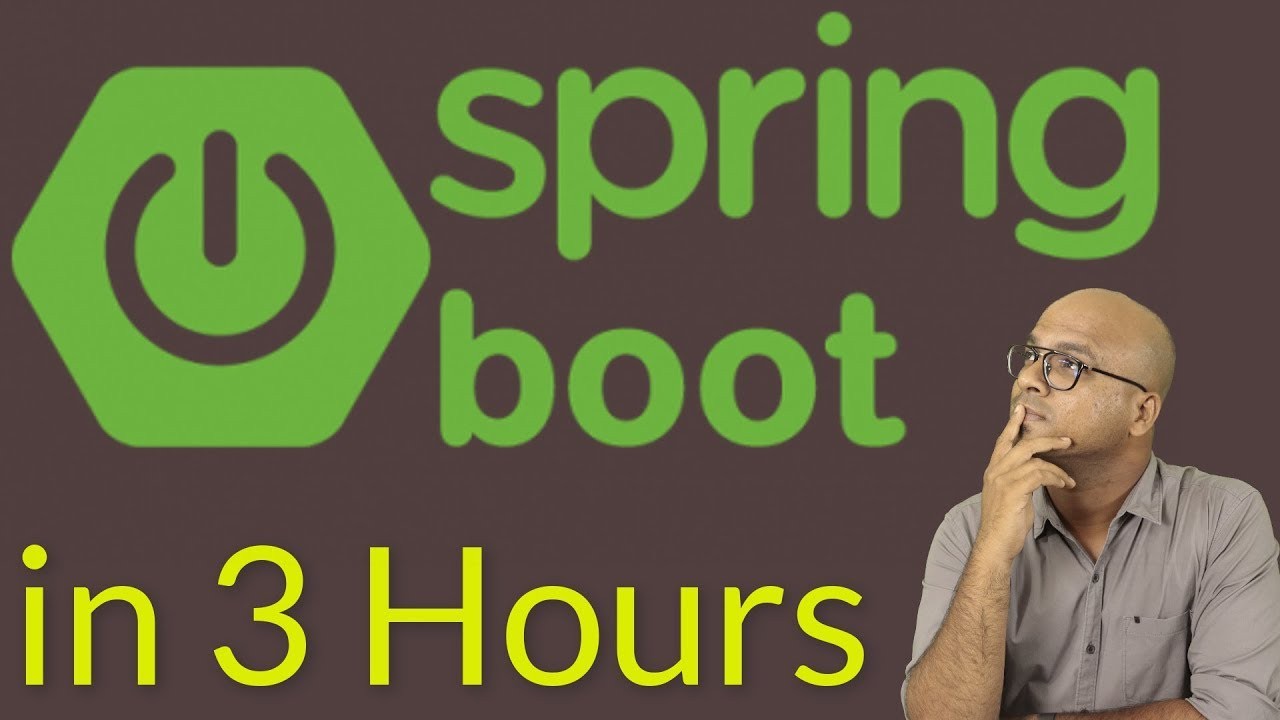
3.2. Tutorials
Tutorials offer a hands-on approach to learning Spring Boot. Here are some popular options:
Tutorial Name | Provider | Description |
---|---|---|
Spring Boot Tutorial | Baeldung | A collection of comprehensive tutorials covering various aspects of Spring Boot, from basic concepts to advanced topics. |
Spring Boot Tutorial for Beginners | TutorialsPoint | A step-by-step guide for beginners, covering the basics of Spring Boot and how to create your first Spring Boot application. |
Spring Boot Tutorial | Udemy | An online course that provides an interactive and structured learning experience, covering the core concepts of Spring Boot. |
Spring Boot REST API Tutorial for Beginners | Java Brains | This tutorial guides beginners through the process of building RESTful APIs with Spring Boot, covering topics like request mapping and data serialization. |
Official Spring Boot Documentation | Spring.io | The official documentation provides comprehensive information on all aspects of Spring Boot, including configuration, features, and best practices. |
3.3. Online Courses
Online courses provide a more immersive and interactive learning experience. Here are some highly rated options:
Course Name | Platform | Description |
---|---|---|
Spring Boot: Beginner to Guru | Udemy | A comprehensive course that covers the entire Spring Boot ecosystem, from basic concepts to advanced topics. |
Spring Boot Fundamentals | Coursera | A free course offered by Google that provides an introduction to Spring Boot and its key features. |
Spring Boot Master Class | Udemy | A hands-on course that covers advanced topics such as Spring Data JPA, Spring Security, and Spring Cloud. |
Learning Spring Boot 2 | LinkedIn Learning | This course provides a comprehensive overview of Spring Boot 2, covering topics like auto-configuration, data access, and microservices. |
RESTful APIs with Spring Boot | Pluralsight | This course focuses on building RESTful APIs with Spring Boot, covering topics like request mapping, data validation, and exception handling. |
3.4. Community Forums
Community forums provide a valuable platform for asking questions, sharing knowledge, and connecting with other Spring Boot users. Here are some active and supportive forums:
Forum Name | URL | Description |
---|---|---|
Spring Boot Forum | https://stackoverflow.com/questions/tagged/spring-boot | The official Spring Boot forum where you can ask questions and get help from the Spring Boot community. |
Stack Overflow | https://spring.io/ | A general programming forum where you can find answers to a wide range of Spring Boot-related questions. |
Reddit Spring Boot | https://www.reddit.com/r/springboot/ | A subreddit dedicated to Spring Boot where you can ask questions, share tips, and discuss the latest news and updates. |
Spring Community | https://spring.io/community | Connect with Spring experts and enthusiasts from around the world. |
GitHub Discussions | https://github.com/spring-projects/spring-boot/discussions | Engage in discussions, ask questions, and share your experiences with Spring Boot on GitHub. |
4. Project-Based Learning: Apply Your Skills
Mastering Spring Boot requires hands-on experience, and project-based learning provides an effective approach to reinforce your understanding. By working on real-world projects, you can apply your knowledge in practical scenarios and develop essential skills. LEARNS.EDU.VN offers a range of project-based courses that allow you to build real-world applications while learning Spring Boot.
4.1. Choosing Suitable Projects
Selecting appropriate projects is crucial. Start with smaller projects that cover basic concepts, then gradually progress to more complex ones. Consider projects that align with your interests and career goals. Open-source projects on platforms like GitHub offer a wealth of options.
Project Idea | Description |
---|---|
Simple RESTful API | Build a basic RESTful API for managing a collection of books, products, or users. |
To-Do List Application | Create a web application for managing a to-do list, with features like adding, deleting, and marking tasks as complete. |
Blog Engine | Develop a simple blog engine with features like creating, editing, and publishing blog posts. |
E-commerce Application | Build an e-commerce application with features like product catalog, shopping cart, and checkout process. |
Social Media Application | Create a social media application with features like user profiles, posts, comments, and likes. |
4.2. Structuring and Managing Projects
Organize your projects efficiently using version control systems like Git. Create clear project structures with well-defined packages and classes. Utilize project management tools like Maven or Gradle to automate build processes and dependency management. Regular testing and documentation ensure project quality and maintainability.
Project Management Task | Description |
---|---|
Version Control | Use Git to track changes to your code, collaborate with others, and revert to previous versions if needed. |
Project Structure | Organize your code into logical packages and classes, following best practices for code organization and maintainability. |
Dependency Management | Use Maven or Gradle to manage your project’s dependencies, ensuring that you have the correct versions of libraries and frameworks. |
Testing | Write unit tests and integration tests to ensure that your code works as expected and to catch bugs early. |
Documentation | Document your code with comments and create a README file to explain how to set up and run your project. |
4.3. Contributing to Open Source Projects
Contributing to open-source projects is an excellent way to improve your Spring Boot skills and gain real-world experience. Find projects on platforms like GitHub that align with your interests and contribute code, documentation, or bug fixes.
Open Source Project | Description |
---|---|
Spring Boot | Contribute to the Spring Boot framework itself, helping to improve its features, fix bugs, and add new functionality. |
Spring Data JPA | Contribute to the Spring Data JPA project, which simplifies data access in Spring Boot applications. |
Micrometer | Contribute to Micrometer, a metrics collection facade for Java virtual machine-based applications. |
Resilience4j | Contribute to Resilience4j, a fault tolerance library inspired by Netflix Hystrix. |
5. Troubleshooting and Debugging: Resolving Issues
Spring Boot applications are generally robust, but errors can still occur. Troubleshooting and debugging are essential skills for any developer. LEARNS.EDU.VN provides resources and support to help you effectively troubleshoot and debug your Spring Boot applications.
5.1. Common Troubleshooting Techniques
Common troubleshooting techniques include:
- Inspecting the log files: Spring Boot generates detailed log files that can help you identify the source of errors.
- Using a debugger: Use a debugger to step through the code, set breakpoints, and examine variables.
- Examining the stack trace: The stack trace provides a detailed list of method calls that led to the error.
- Consulting documentation and online resources: Refer to the Spring Boot documentation, online forums, and Stack Overflow for solutions to common problems.
Troubleshooting Technique | Description |
---|---|
Log File Inspection | Examine the log files for error messages, warnings, and debugging information that can help you identify the cause of the problem. |
Debugger Usage | Use a debugger to step through your code line by line, inspect variables, and identify the point where the error occurs. |
Stack Trace Analysis | Analyze the stack trace to understand the sequence of method calls that led to the error and identify the source of the exception. |
Documentation Review | Consult the Spring Boot documentation and online resources for solutions to common problems and best practices. |
5.2. Spring Boot Debugging Tools
Spring Boot provides several debugging tools:
- Spring Boot DevTools: Provides automatic application restarts, live reloading, and other development-time features.
- Spring Boot Actuator: Provides endpoints for monitoring and managing your application.
- Spring Boot JMX: Provides a JMX interface for monitoring and managing your application.
Debugging Tool | Description |
---|---|
Spring Boot DevTools | Automatically restarts your application when changes are detected, allowing you to quickly test your code without manually restarting. |
Spring Boot Actuator | Provides endpoints for monitoring your application’s health, metrics, and configuration. |
Spring Boot JMX | Exposes your application’s configuration and metrics through a JMX interface, allowing you to monitor and manage it remotely. |
5.3. Common Errors and Solutions
Common errors in Spring Boot applications include:
- Configuration errors: Errors in the
application.properties
orapplication.yml
file. - Bean definition errors: Errors in the Spring configuration, such as missing or ambiguous bean definitions.
- Dependency conflicts: Conflicts between different versions of libraries.
- Runtime exceptions: Exceptions that occur during the execution of your code.
Error Type | Description |
---|---|
Configuration Errors | Occur when there are mistakes in your application’s configuration files, such as incorrect database URLs or missing properties. |
Bean Definition Errors | Occur when there are problems with the Spring configuration, such as missing or ambiguous bean definitions. |
Dependency Conflicts | Occur when there are conflicts between different versions of libraries, leading to unexpected behavior or runtime exceptions. |
Runtime Exceptions | Occur during the execution of your code, such as NullPointerExceptions or IOExceptions. |
By understanding these common errors and using the appropriate troubleshooting techniques, developers can quickly identify and resolve issues in their Spring Boot applications.
6. Advanced Topics and Best Practices for Spring Boot Mastery
Spring Boot offers a comprehensive set of advanced features and best practices to enhance the development and maintenance of enterprise-grade applications. LEARNS.EDU.VN provides in-depth courses covering these advanced topics to help you become a Spring Boot expert.
6.1. Spring Data JPA: Simplify Data Access
Spring Data JPA provides a powerful abstraction layer for working with relational databases. It simplifies data access, reduces boilerplate code, and enables seamless integration with Spring Boot’s core features.
- Simplifies CRUD operations through repository interfaces.
- Supports pagination, sorting, and filtering.
- Enables automatic schema generation and database initialization.
Feature | Description |
---|---|
Repository Interfaces | Provides a simple and consistent way to access data, reducing the amount of boilerplate code required for CRUD operations. |
Pagination and Sorting | Supports pagination and sorting of data, making it easy to retrieve large datasets in manageable chunks. |
Automatic Schema Generation | Automatically generates database schemas based on your entity definitions, simplifying database setup and maintenance. |
6.2. RESTful APIs: Build Web Services
Spring Boot makes it easy to create RESTful APIs with its built-in support for JSON and XML serialization, HTTP request mapping, and exception handling.
- Simplifies the creation of REST controllers.
- Provides annotations for request mapping and parameter validation.
- Offers support for content negotiation and versioning.
Feature | Description |
---|---|
REST Controllers | Provides a simple way to create RESTful endpoints using annotations like @RestController , @RequestMapping , and @GetMapping . |
Request Mapping | Allows you to map HTTP requests to specific controller methods based on URL patterns and HTTP methods. |
Content Negotiation | Supports content negotiation, allowing clients to request data in different formats like JSON or XML. |
6.3. Spring Security: Secure Your Application
Spring Security provides robust authentication and authorization mechanisms for Spring Boot applications. It supports various authentication providers, authorization strategies, and security filters.
- Protects applications from unauthorized access.
- Offers support for OAuth2, JWT, and LDAP authentication.
- Provides granular access control through role-based authorization.
Feature | Description |
---|---|
Authentication | Provides mechanisms for verifying the identity of users, such as username/password authentication, OAuth2, and JWT. |
Authorization | Provides mechanisms for controlling access to resources based on user roles or permissions. |
Access Control Lists | Allows you to define granular access control rules for individual objects or resources. |
7. Career Opportunities and Industry Use Cases for Spring Boot Developers
Spring Boot developers are in high demand due to the framework’s popularity and wide adoption. They can work in various industries, including software development, financial services, healthcare, and e-commerce. Spring Boot’s ease of use, flexibility, and scalability make it an ideal choice for building enterprise-grade applications. Developers can create complex systems with minimal effort, saving time and resources. LEARNS.EDU.VN provides career guidance and job placement assistance to help you launch your Spring Boot development career.
7.1. Job Roles for Spring Boot Developers
Spring Boot developers can pursue various job roles, including:
Job Role | Description |
---|---|
Java Developer | Develop and maintain Java-based applications using Spring Boot. |
Spring Boot Developer | Specialize in developing Spring Boot applications, focusing on backend development and API design. |
Full Stack Developer | Develop both frontend and backend components of web applications using Spring Boot and related technologies. |
Microservices Architect | Design and implement microservices architectures using Spring Boot and Spring Cloud. |
Cloud Engineer | Deploy and manage Spring Boot applications on cloud platforms like AWS, Azure, and Google Cloud. |
7.2. Industries Hiring Spring Boot Developers
Spring Boot developers are in demand across various industries, including:
Industry | Description |
---|---|
Financial Services | Build banking applications, payment systems, and trading platforms. |
Healthcare | Develop electronic health records (EHR) systems, patient portals, and medical devices. |
E-commerce | Build online stores, shopping carts, and payment gateways. |
Education | Develop learning management systems (LMS), student portals, and educational games. |
Government | Build government websites, citizen portals, and tax systems. |
7.3. Salary Expectations for Spring Boot Developers
The salary expectations for Spring Boot developers vary based on experience, skills, and location. According to Glassdoor, the average salary for a Spring Boot developer in the United States is around $110,000 per year.
Experience Level | Average Salary (USD) |
---|---|
Entry-Level | $80,000 – $90,000 |
Mid-Level | $100,000 – $120,000 |
Senior-Level | $130,000 – $150,000+ |
8. Spring Boot in Action: Detailed Industry Use Cases
Spring Boot is used in a wide range of industries to build various applications. Here are some detailed industry use cases:
8.1. Financial Services
Spring Boot is used in the financial services industry to build banking applications, payment systems, and trading platforms.
- Banking Applications: Spring Boot is used to build online banking applications, mobile banking apps, and core banking systems.
- Payment Systems: Spring Boot is used to build payment gateways, payment processing systems, and fraud detection systems.
- Trading Platforms: Spring Boot is used to build trading platforms, market data feeds, and risk management systems.
8.2. Healthcare
Spring Boot is used in the healthcare industry to develop electronic health records (EHR) systems, patient portals, and medical devices.
- Electronic Health Records (EHR) Systems: Spring Boot is used to build EHR systems that store and manage patient medical records.
- Patient Portals: Spring Boot is used to build patient portals that allow patients to access their medical records, schedule appointments, and communicate with their healthcare providers.
- Medical Devices: Spring Boot is used to develop software for medical devices, such as pacemakers, insulin pumps, and diagnostic equipment.
8.3. E-commerce
Spring Boot is used in the e-commerce industry to build online stores, shopping carts, and payment gateways.
- Online Stores: Spring Boot is used to build online stores that allow customers to browse products, add items to their cart, and place orders.
- Shopping Carts: Spring Boot is used to build shopping cart systems that track the items that customers have added to their cart.
- Payment Gateways: Spring Boot is used to build payment gateways that process credit card payments and other forms of payment.
8.4. Education
Spring Boot is used in the education industry to develop learning management systems (LMS), student portals, and educational games.
- Learning Management Systems (LMS): Spring Boot is used to build LMS that allow students to access course materials, submit assignments, and track their progress.
- Student Portals: Spring Boot is used to build student portals that allow students to access their grades, view their transcripts, and register for courses.
- Educational Games: Spring Boot is used to develop educational games that make learning fun and engaging.
8.5. Government
Spring Boot is used in the government sector to build government websites, citizen portals, and tax systems.
- Government Websites: Spring Boot is used to build government websites that provide information about government services, policies, and regulations.
- Citizen Portals: Spring Boot is used to build citizen portals that allow citizens to access government services online, such as applying for permits, paying taxes, and registering to vote.
- Tax Systems: Spring Boot is used to build tax systems that process tax returns and calculate tax liabilities.
9. FAQs: Addressing Your Questions About Learning Spring Boot
Here are some frequently asked questions about learning Spring Boot:
9.1. How long does it take to learn Spring Boot?
The learning timeline varies based on your prior Java knowledge and the complexity of projects you undertake. With consistent effort, you can become proficient within a few months.
9.2. What resources are essential for learning Spring Boot?
Spring Boot’s official documentation, tutorials, online courses, and community forums provide a wealth of knowledge and support.
9.3. How can I enhance my Spring Boot skills?
Project-based learning is crucial. Choose projects that challenge you and provide opportunities to apply your knowledge in practical scenarios.
9.4. Is Spring Boot difficult to learn?
Spring Boot can be challenging for beginners, but with a solid foundation in Java and a structured learning approach, it becomes manageable.
9.5. What are the prerequisites for learning Spring Boot?
The main prerequisites are a good understanding of Java, basic knowledge of web development, and familiarity with build tools like Maven or Gradle.
9.6. Can I learn Spring Boot without knowing Spring?
While knowledge of Spring is helpful, it’s not strictly necessary. Spring Boot simplifies the configuration and setup process, making it easier to get started.
9.7. What are the key concepts to learn in Spring Boot?
Key concepts include auto-configuration, dependency injection, Spring Data JPA, RESTful APIs, and Spring Security.
9.8. How do I stay updated with the latest Spring Boot features?
Follow the official Spring Boot blog, attend conferences, and participate in community forums to stay informed about the latest features and updates.
9.9. What are some common mistakes to avoid when learning Spring Boot?
Common mistakes include neglecting the fundamentals, skipping documentation, and not practicing enough.
9.10. How can LEARNS.EDU.VN help me learn Spring Boot?
LEARNS.EDU.VN provides comprehensive courses, personalized learning paths, expert guidance, and project-based learning opportunities to help you master Spring Boot efficiently.
10. Conclusion: Your Journey to Spring Boot Mastery Starts Now
Learning Spring Boot can open up a world of opportunities in the software development industry. While the timeline for mastering Spring Boot depends on various factors, with dedication, consistent practice, and the right resources, you can achieve your goals. LEARNS.EDU.VN is here to support you on your journey, providing the knowledge, skills, and guidance you need to succeed. Visit LEARNS.EDU.VN today to explore our comprehensive Spring Boot courses and start your path to becoming a proficient Spring Boot developer.
Ready to take your skills to the next level? Explore the comprehensive Spring Boot courses available at LEARNS.EDU.VN. Whether you’re a beginner or an experienced developer, our expert-led courses offer hands-on training and real-world projects to help you master Spring Boot. Visit LEARNS.EDU.VN, located at 123 Education Way, Learnville, CA 90210, United States, or contact us via WhatsApp at +1 555-555-1212 to learn more. Let learns.edu.vn guide you on your journey to becoming a proficient Spring Boot developer.