Learning How To Learn Scripting In Roblox Studio opens up a world of creative possibilities, allowing you to design immersive and engaging experiences. At LEARNS.EDU.VN, we guide you through the process of mastering Luau, Roblox’s scripting language, and help you bring your game ideas to life with practical examples and step-by-step tutorials. Discover effective methods and resources to become proficient in game development and elevate your skills with scripting techniques for Roblox Studio, empowering your game creation journey.
1. Understanding the Basics of Roblox Scripting
Before diving into the specifics of scripting, it’s important to understand the fundamental concepts. Scripting in Roblox Studio revolves around the Luau programming language, a fast and efficient language designed for game development. According to Roblox’s official documentation, Luau combines the best aspects of Lua with additional features to enhance performance and security.
- What is Luau? Luau is the scripting language used in Roblox Studio. It’s derived from Lua but includes optimizations and features specific to Roblox.
- Objects and Instances: Everything in Roblox is an object. Parts, models, scripts, and even the game itself are all instances of various classes.
- Properties: Objects have properties that define their characteristics, such as color, size, position, and behavior.
- Events: Events are actions that occur in the game, such as a player joining, a part being touched, or a value changing.
- Functions: Functions are blocks of code that perform specific tasks. They can be called multiple times and can accept arguments to customize their behavior.
Understanding these basics is crucial for effectively learning and applying scripting in Roblox Studio.
2. Setting Up Your Roblox Studio Environment
To start learning scripting, you’ll need to set up your Roblox Studio environment. This involves downloading and installing Roblox Studio, familiarizing yourself with the interface, and creating a new project.
- Download and Install Roblox Studio:
- Go to the Roblox website and create an account if you don’t already have one.
- Download Roblox Studio from the “Create” section of the website.
- Install Roblox Studio on your computer, following the on-screen instructions.
- Familiarize Yourself with the Interface:
- The Roblox Studio interface consists of several panels:
- Explorer: Shows the hierarchy of objects in your game.
- Properties: Allows you to modify the properties of selected objects.
- Toolbox: Provides access to models, images, and other assets.
- Output: Displays messages, errors, and debugging information.
- Viewport: The 3D view where you build and test your game.
- The Roblox Studio interface consists of several panels:
- Create a New Project:
- Open Roblox Studio and choose a template or create a new baseplate.
- Save your project to a location on your computer.
With your environment set up, you’re ready to start writing your first scripts.
3. Writing Your First Script: A Step-by-Step Guide
Let’s walk through writing a simple script that changes the color of a part in Roblox Studio. This will help you understand the basic syntax and structure of Luau.
-
Insert a Part:
- In the Explorer window, right-click on “Workspace” and select “Insert Object.”
- Choose “Part” from the list of objects.
- Rename the part to “ColorChanger.”
-
Insert a Script:
- Right-click on “ColorChanger” in the Explorer window and select “Insert Object.”
- Choose “Script” from the list of objects.
- Rename the script to “ColorChangeScript.”
-
Write the Script:
- Open the script by double-clicking on “ColorChangeScript” in the Explorer window.
- Replace the default code with the following:
local part = script.Parent part.Touched:Connect(function(hit) if hit.Parent:FindFirstChild("Humanoid") then part.BrickColor = BrickColor.random() end end)
-
Explanation of the Script:
local part = script.Parent
assigns the part that the script is parented to (ColorChanger) to the variablepart
.part.Touched:Connect(function(hit))
connects a function to theTouched
event of the part. This event fires whenever the part is touched by another object.if hit.Parent:FindFirstChild("Humanoid") then
checks if the object that touched the part is a player (by checking if it has a Humanoid object).part.BrickColor = BrickColor.random()
changes the color of the part to a random color.
-
Test the Script:
- Click the “Play” button in Roblox Studio to start the game.
- Walk your character into the “ColorChanger” part.
- The part should change color randomly each time you touch it.
This simple script demonstrates the basic structure of a Roblox script and how to interact with objects and events.
4. Essential Scripting Concepts in Roblox
To become proficient in Roblox scripting, you’ll need to master several essential concepts. These include variables, data types, control structures, functions, and events.
4.1 Variables and Data Types
Variables are used to store data in your scripts. In Luau, you can declare variables using the local
keyword. There are several data types in Luau, including:
- Number: Represents numeric values, such as integers and decimals (e.g.,
10
,3.14
). - String: Represents text (e.g.,
"Hello, World!"
). - Boolean: Represents true or false values (e.g.,
true
,false
). - Nil: Represents the absence of a value (e.g.,
nil
). - Table: Represents a collection of key-value pairs (e.g.,
{name = "John", age = 30}
).
Example:
local playerName = "JohnDoe" -- String variable
local playerScore = 100 -- Number variable
local isGameOver = false -- Boolean variable
local inventory = {} -- Table variable
4.2 Control Structures
Control structures allow you to control the flow of your code. The most common control structures are:
- If Statements: Execute a block of code only if a certain condition is true.
- While Loops: Execute a block of code repeatedly as long as a certain condition is true.
- For Loops: Execute a block of code a specific number of times or iterate over a collection of items.
Example of an If Statement:
local score = 75
if score >= 60 then
print("Passed")
else
print("Failed")
end
Example of a While Loop:
local count = 0
while count < 10 do
print("Count:", count)
count = count + 1
end
Example of a For Loop:
local colors = {"Red", "Green", "Blue"}
for i, color in ipairs(colors) do
print("Color", i, ":", color)
end
4.3 Functions
Functions are reusable blocks of code that perform specific tasks. They can accept arguments and return values.
Example:
local function add(a, b)
return a + b
end
local sum = add(5, 3) -- sum will be 8
print(sum)
4.4 Events
Events are actions that occur in the game. You can connect functions to events to respond to these actions.
Example:
local part = script.Parent
part.Touched:Connect(function(hit)
print("Part touched by:", hit.Name)
end)
This script prints the name of the object that touched the part to the output window.
5. Advanced Scripting Techniques
Once you have a solid understanding of the basics, you can move on to more advanced scripting techniques. These include:
- Object-Oriented Programming (OOP): A programming paradigm that uses objects to represent data and behavior.
- Remote Events and Functions: Allow scripts on the client (player’s computer) to communicate with scripts on the server (Roblox’s servers).
- Data Persistence: Saving and loading data, such as player scores and inventory, using DataStoreService.
- Animation: Creating and controlling animations for characters and objects.
- Artificial Intelligence (AI): Implementing AI behaviors for non-player characters (NPCs).
5.1 Object-Oriented Programming (OOP)
OOP is a powerful programming paradigm that allows you to organize your code into reusable and modular objects. In Roblox, you can use OOP to create classes and objects that represent different entities in your game.
Example:
local Character = {}
Character.__index = Character
function Character.new(name, health)
local self = setmetatable({}, Character)
self.Name = name
self.Health = health
return self
end
function Character:TakeDamage(damage)
self.Health = self.Health - damage
print(self.Name .. " took " .. damage .. " damage. Health: " .. self.Health)
end
local player = Character.new("John", 100)
player:TakeDamage(20) -- Output: John took 20 damage. Health: 80
5.2 Remote Events and Functions
Remote events and functions allow you to communicate between the client and the server. This is essential for creating multiplayer games and handling sensitive data on the server.
Example:
Server Script:
local remoteEvent = Instance.new("RemoteEvent")
remoteEvent.Name = "UpdateScore"
remoteEvent.Parent = game:GetService("ReplicatedStorage")
remoteEvent.OnServerEvent:Connect(function(player, score)
print(player.Name .. "'s score updated to " .. score)
end)
Client Script:
local remoteEvent = game:GetService("ReplicatedStorage"):WaitForChild("UpdateScore")
remoteEvent:FireServer(150) -- Send the score to the server
5.3 Data Persistence
Data persistence allows you to save and load data, such as player scores, inventory, and settings. This is essential for creating games with progression and long-term engagement.
Example:
local DataStoreService = game:GetService("DataStoreService")
local playerDataStore = DataStoreService:GetDataStore("PlayerData")
game.Players.PlayerAdded:Connect(function(player)
local success, data = pcall(function()
return playerDataStore:GetAsync(player.UserId)
end)
if success and data then
-- Load player data
player.leaderstats.Score.Value = data.Score
else
-- Create default data
player.leaderstats.Score.Value = 0
end
end)
game.Players.PlayerRemoving:Connect(function(player)
local data = {
Score = player.leaderstats.Score.Value
}
local success, errorMessage = pcall(function()
playerDataStore:SetAsync(player.UserId, data)
end)
if not success then
warn("Error saving data: " .. errorMessage)
end
end)
5.4 Animation
Animation allows you to bring your characters and objects to life with movement and expressions. Roblox provides tools and APIs for creating and controlling animations.
Example:
local humanoid = script.Parent:WaitForChild("Humanoid")
local animation = script:WaitForChild("Animation")
local animationTrack = humanoid:LoadAnimation(animation)
animationTrack:Play()
5.5 Artificial Intelligence (AI)
AI allows you to create intelligent behaviors for non-player characters (NPCs). This can include pathfinding, combat, and decision-making.
Example:
local PathfindingService = game:GetService("PathfindingService")
local npc = script.Parent
local humanoid = npc:WaitForChild("Humanoid")
local function MoveTo(destination)
local path = PathfindingService:CreatePath(npc)
path:ComputeAsync(npc.PrimaryPart.Position, destination)
if path.Status == Enum.PathStatus.Success then
for i, waypoint in ipairs(path:GetWaypoints()) do
humanoid:MoveTo(waypoint.Position)
humanoid.MoveToFinished:Wait()
end
end
end
-- Example usage
MoveTo(Vector3.new(10, 0, 20))
6. Tips for Effective Learning
Learning Roblox scripting can be challenging, but with the right approach, you can make the process more efficient and enjoyable. Here are some tips for effective learning:
- Start with the Basics: Don’t try to learn everything at once. Focus on mastering the fundamental concepts before moving on to more advanced topics.
- Practice Regularly: The best way to learn scripting is to practice. Write code every day, even if it’s just for a few minutes.
- Work on Projects: Apply your knowledge by working on small projects. This will help you understand how different concepts fit together and give you a sense of accomplishment.
- Read Documentation: Roblox’s official documentation is a valuable resource. Refer to it often to learn about different objects, properties, events, and functions.
- Join the Community: Connect with other Roblox developers in online forums, Discord servers, and social media groups. Ask questions, share your work, and learn from others.
- Watch Tutorials: There are many excellent video tutorials available on YouTube and other platforms. Watch tutorials to learn new techniques and see how others approach scripting challenges.
- Deconstruct Games: If you find a game on Roblox that you admire, try to deconstruct it. Analyze the scripts and see how they work. This can give you valuable insights into game development techniques.
- Debug Your Code: Learning to debug your code is essential. Use print statements and Roblox Studio’s debugging tools to identify and fix errors.
- Stay Patient: Learning scripting takes time and effort. Don’t get discouraged if you encounter challenges. Keep practicing and learning, and you’ll eventually master the skills you need to create amazing games.
7. Resources for Learning Roblox Scripting
There are many resources available to help you learn Roblox scripting. Here are some of the most popular and effective resources:
Resource | Description | URL |
---|---|---|
Roblox Developer Hub | Official documentation with comprehensive information on all aspects of Roblox development. | https://create.roblox.com/ |
Roblox Creator Hub | A platform containing various resources, tutorials, and guides to help creators succeed in Roblox game development. | https://create.roblox.com/creations |
YouTube Tutorials | Numerous channels offer tutorials on Roblox scripting, ranging from beginner to advanced levels. | Search “Roblox Scripting Tutorial” on YouTube |
Udemy Courses | Paid courses that provide structured learning paths and in-depth coverage of Roblox scripting topics. | https://www.udemy.com/ (Search “Roblox Scripting”) |
Roblox Developer Forum | A community forum where you can ask questions, share your work, and get feedback from other developers. | https://devforum.roblox.com/ |
LEARNS.EDU.VN | A website providing educational content, including tutorials and courses on Roblox scripting, designed to help learners of all levels. | LEARNS.EDU.VN |
Roblox API Reference | Detailed documentation of Roblox’s API, including objects, properties, events, and functions. | https://create.roblox.com/docs/reference |
AlvinBlox (YouTube Channel) | A popular YouTube channel with a wide range of Roblox scripting tutorials for beginners and advanced users. | https://www.youtube.com/@AlvinBlox |
TheDevKing (YouTube Channel) | Another popular YouTube channel offering comprehensive Roblox scripting tutorials and game development tips. | https://www.youtube.com/@TheDevKing |
ClearCode (YouTube Channel) | Provides clear and concise tutorials on Roblox scripting, focusing on specific topics and techniques. | https://www.youtube.com/@ClearCodeYT |
RoDevs (Discord Community) | A Discord server dedicated to Roblox development, where you can connect with other developers, ask questions, and get help with your projects. | Search “RoDevs Discord” on Discord |
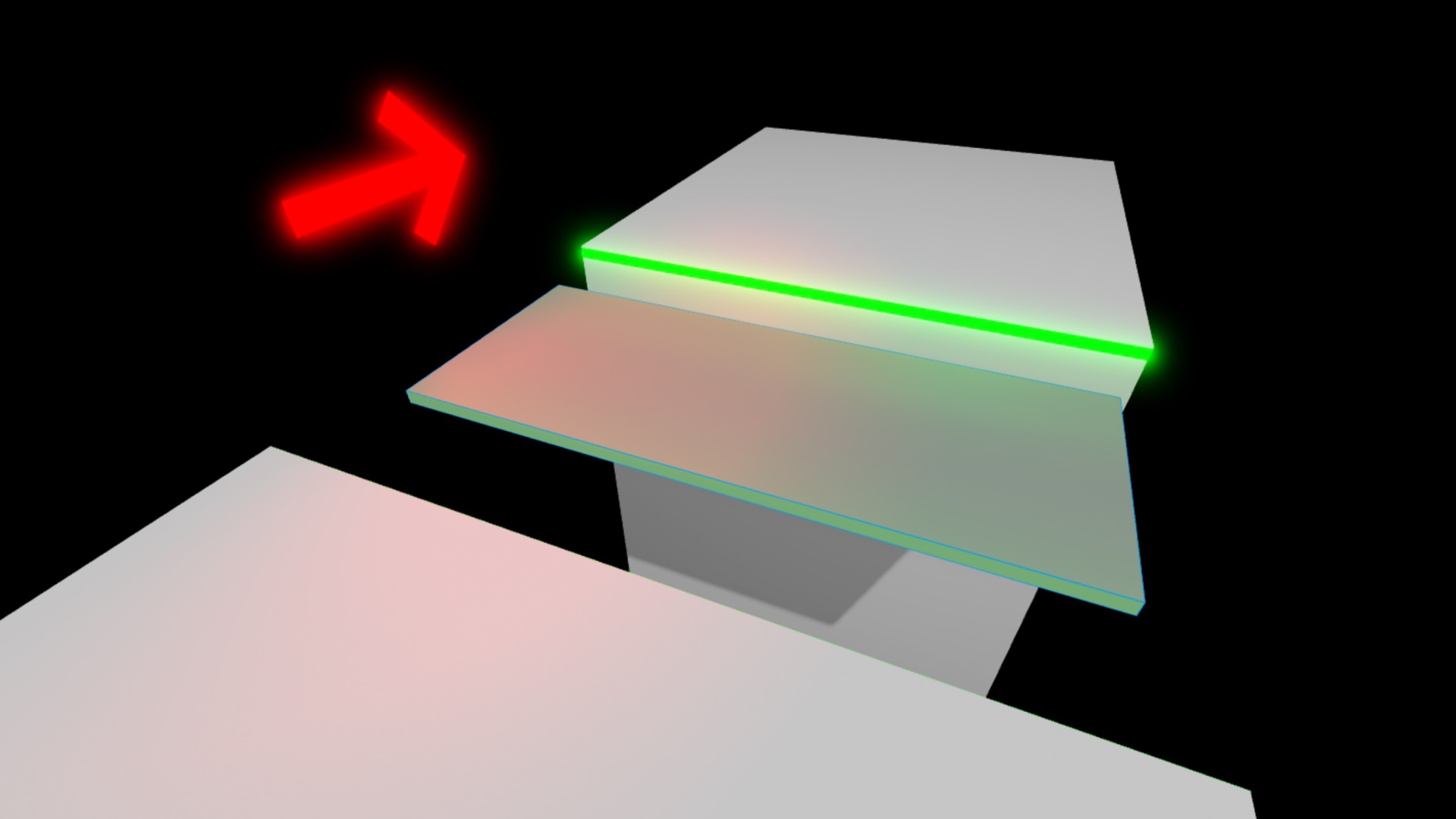
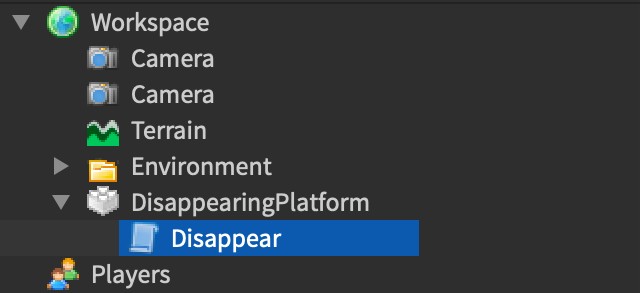
8. Common Mistakes to Avoid
When learning Roblox scripting, it’s easy to make mistakes. However, by being aware of these common pitfalls, you can avoid them and accelerate your learning process.
- Not Understanding the Basics: Trying to jump into advanced topics without a solid understanding of the basics.
- Ignoring Errors: Not paying attention to error messages and warnings in the output window.
- Copying Code Without Understanding: Copying and pasting code without understanding how it works.
- Not Commenting Your Code: Failing to add comments to explain your code.
- Not Testing Your Code: Not testing your code frequently and thoroughly.
- Overcomplicating Things: Trying to solve problems with overly complex solutions.
- Not Asking for Help: Being afraid to ask for help when you’re stuck.
- Not Using Version Control: Not using version control to track changes to your code.
- Not Optimizing Your Code: Writing inefficient code that can cause performance problems.
- Not Staying Up-to-Date: Not keeping up with the latest updates and changes to the Roblox API.
9. Practical Examples and Projects
To solidify your understanding of Roblox scripting, it’s helpful to work on practical examples and projects. Here are some ideas for projects that you can try:
- Simple Obby: Create a simple obstacle course with moving platforms, traps, and checkpoints.
- Clicker Game: Develop a clicker game where players earn points by clicking on an object.
- Tycoon Game: Build a tycoon game where players earn money and expand their base.
- Fighting Game: Create a fighting game with different characters, moves, and abilities.
- Role-Playing Game (RPG): Develop an RPG with quests, characters, and a storyline.
- Survival Game: Build a survival game where players must gather resources, craft items, and defend themselves against enemies.
- Puzzle Game: Create a puzzle game with logic puzzles, mazes, and riddles.
- Racing Game: Develop a racing game with different cars, tracks, and power-ups.
- Tower Defense Game: Build a tower defense game where players must defend their base against waves of enemies.
- Simulation Game: Create a simulation game where players can simulate real-world activities, such as farming, building, or managing a business.
9.1 Creating a Disappearing Platform
Let’s revisit the example from the original article and expand on it to create a more sophisticated disappearing platform.
-
Set Up the Scene:
- Insert a Part and rename it to “DisappearingPlatform.”
- Resize it to be large enough for a player to jump on.
- Move it to a location where the player can reach it.
- Set the Anchored property to true.
-
Insert a Script:
- Insert a Script into the “DisappearingPlatform” and rename it to “DisappearScript.”
- Replace the default code with the following:
local platform = script.Parent local isVisible = true local disappearTime = 3 local appearTime = 5 local function disappear() platform.CanCollide = false platform.Transparency = 1 isVisible = false end local function appear() platform.CanCollide = true platform.Transparency = 0 isVisible = true end while true do if isVisible then task.wait(disappearTime) disappear() else task.wait(appearTime) appear() end end
-
Customize the Script:
- Adjust the
disappearTime
andappearTime
variables to change how long the platform is visible and invisible. - Add sound effects when the platform disappears and reappears.
- Make the platform flash before disappearing to give players a warning.
- Implement a system where the platform only disappears when a player is standing on it.
- Adjust the
9.2 Adding Sound Effects
To add sound effects, you can use the Sound
object in Roblox Studio.
-
Insert a Sound:
- Insert a Sound object into the “DisappearingPlatform.”
- Rename it to “DisappearSound.”
- Set the SoundId property to the ID of a sound effect from the Roblox library.
-
Modify the Script:
- Add the following code to the
disappear
function:
local sound = platform:WaitForChild("DisappearSound") sound:Play()
- Add the following code to the
-
Repeat for Appear Sound:
- Create another sound object called “AppearSound” and add similar code to the
appear
function.
- Create another sound object called “AppearSound” and add similar code to the
9.3 Implementing a Warning Flash
To make the platform flash before disappearing, you can use a for
loop to change the transparency of the platform rapidly.
-
Modify the Script:
- Add the following code before the
disappear
function call:
for i = 1, 5 do platform.Transparency = 0.5 task.wait(0.2) platform.Transparency = 0 task.wait(0.2) end
This code will make the platform flash five times before disappearing.
- Add the following code before the
10. Frequently Asked Questions (FAQ)
Here are some frequently asked questions about learning Roblox scripting:
- What is the best way to learn Roblox scripting? The best way to learn Roblox scripting is to start with the basics, practice regularly, work on projects, read documentation, and join the community.
- Do I need to know programming to learn Roblox scripting? No, you don’t need to know programming to start learning Roblox scripting. However, having some programming experience can be helpful.
- What is Luau? Luau is the scripting language used in Roblox Studio. It’s derived from Lua but includes optimizations and features specific to Roblox.
- How long does it take to learn Roblox scripting? The time it takes to learn Roblox scripting varies depending on your learning style, dedication, and goals. However, with consistent effort, you can learn the basics in a few weeks and become proficient in a few months.
- What are the best resources for learning Roblox scripting? The best resources for learning Roblox scripting include Roblox’s official documentation, YouTube tutorials, Udemy courses, the Roblox Developer Forum, and websites like LEARNS.EDU.VN.
- What are some common mistakes to avoid when learning Roblox scripting? Common mistakes to avoid include not understanding the basics, ignoring errors, copying code without understanding, not commenting your code, and not testing your code.
- What are some good projects to work on to practice Roblox scripting? Good projects to work on include simple obbies, clicker games, tycoon games, fighting games, RPGs, survival games, puzzle games, racing games, tower defense games, and simulation games.
- How can I improve the performance of my Roblox scripts? You can improve the performance of your Roblox scripts by optimizing your code, avoiding unnecessary calculations, using efficient data structures, and minimizing network traffic.
- How can I debug my Roblox scripts? You can debug your Roblox scripts by using print statements, Roblox Studio’s debugging tools, and third-party debugging plugins.
- Where can I get help with Roblox scripting? You can get help with Roblox scripting by asking questions on the Roblox Developer Forum, joining Roblox scripting Discord servers, and contacting experienced Roblox developers.
Learning how to learn scripting in Roblox Studio is an exciting journey that can lead to endless creative possibilities. By mastering Luau, understanding essential scripting concepts, and working on practical projects, you can bring your game ideas to life and create immersive experiences for players around the world. Remember to stay patient, practice regularly, and take advantage of the many resources available to help you along the way. With dedication and perseverance, you can become a proficient Roblox developer and achieve your game development goals.
Ready to dive deeper into the world of Roblox scripting? Visit LEARNS.EDU.VN today to explore our comprehensive tutorials and courses designed to elevate your game development skills. Whether you’re a beginner or an experienced coder, learns.edu.vn provides the resources and guidance you need to succeed. Unlock your creative potential and start building amazing games today! Contact us at 123 Education Way, Learnville, CA 90210, United States or Whatsapp: +1 555-555-1212.