What Do You Learn In Cs? Computer Science is a diverse field, and this comprehensive guide from LEARNS.EDU.VN explores the core subjects, skills, and knowledge you’ll gain. Discover how a CS education equips you for a future in tech and beyond, covering fundamental concepts like data structures and algorithms to specialized areas like web development and computer architecture, ultimately enhancing your problem-solving abilities and opening doors to exciting career paths.
1. What is Computer Science and What Do You Learn?
Computer Science (CS) is the study of computers and computational systems. Unlike fields that deal with specific hardware, CS focuses on the underlying principles of computation, algorithm design, programming languages, and information management. It’s a broad discipline with applications in almost every aspect of modern life, from software development and data analysis to artificial intelligence and cybersecurity.
1.1. Understanding the Core Concepts
At the heart of computer science lies a set of fundamental concepts:
- Algorithms: Step-by-step procedures for solving problems. They are the backbone of any computational process.
- Data Structures: Organized ways of storing and manipulating data, such as arrays, linked lists, trees, and graphs.
- Programming Languages: Tools for instructing computers to perform specific tasks, ranging from low-level languages like assembly to high-level languages like Python and Java.
- Computer Architecture: The design and organization of computer systems, including the central processing unit (CPU), memory, and input/output devices.
- Operating Systems: Software that manages computer hardware and provides services for applications.
- Databases: Systems for storing and retrieving large amounts of structured data.
- Networks: Infrastructure for connecting computers and enabling communication.
- Software Engineering: Principles and practices for designing, developing, and maintaining large software systems.
These concepts form the foundation for more specialized areas within computer science.
1.2. Why Study Computer Science?
Studying computer science offers numerous benefits:
- Problem-Solving Skills: CS teaches you how to break down complex problems into smaller, manageable parts and develop logical solutions.
- Analytical Thinking: CS encourages you to analyze data, identify patterns, and draw conclusions based on evidence.
- Creativity and Innovation: CS empowers you to create new technologies and solve real-world problems in innovative ways.
- Career Opportunities: The demand for computer science professionals is high and growing, with a wide range of career paths available.
- Intellectual Stimulation: CS is a challenging and rewarding field that keeps you constantly learning and exploring new ideas.
- Impact on Society: CS allows you to contribute to solving some of the world’s most pressing problems, from climate change and healthcare to education and poverty.
1.3. Core Subjects in a Computer Science Curriculum
A typical computer science curriculum covers a range of subjects designed to provide a solid foundation in the field. Here are some common core courses:
Course | Description |
---|---|
Programming Fundamentals | Introduces basic programming concepts, such as variables, data types, control structures, and functions. |
Data Structures & Algorithms | Covers fundamental data structures (arrays, linked lists, trees, graphs) and algorithms for sorting, searching, and manipulating data. |
Discrete Mathematics | Provides the mathematical foundations for computer science, including logic, set theory, combinatorics, and graph theory. |
Computer Architecture | Explores the design and organization of computer systems, including the CPU, memory, and input/output devices. |
Operating Systems | Covers the principles and design of operating systems, including process management, memory management, and file systems. |
Database Management Systems | Introduces the concepts and techniques for designing, implementing, and managing databases. |
Computer Networks | Explores the principles and protocols of computer networks, including TCP/IP, HTTP, and DNS. |
Software Engineering | Covers the principles and practices for designing, developing, and maintaining large software systems, including requirements analysis, design patterns, and testing methodologies. |
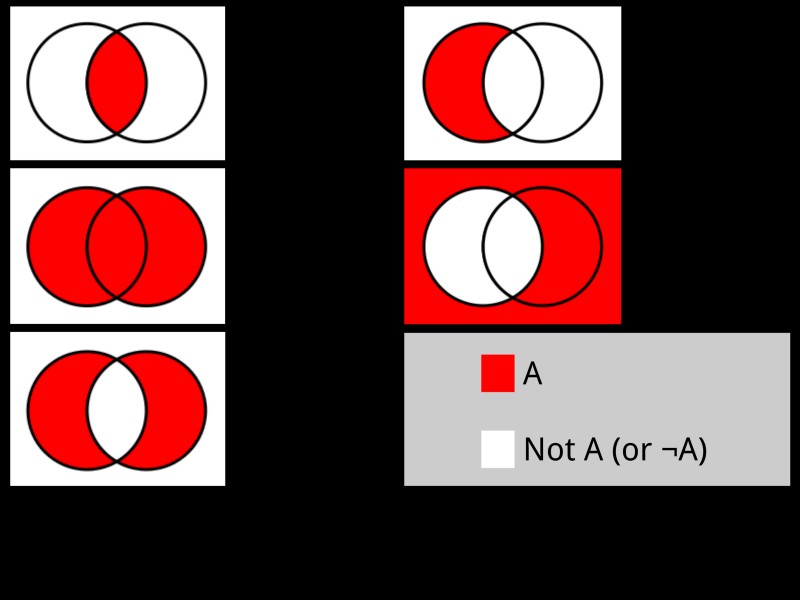
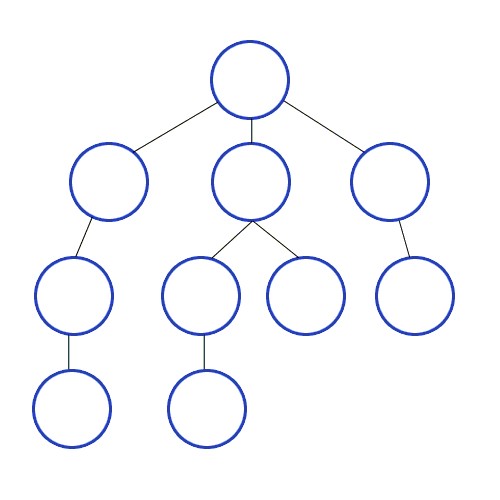
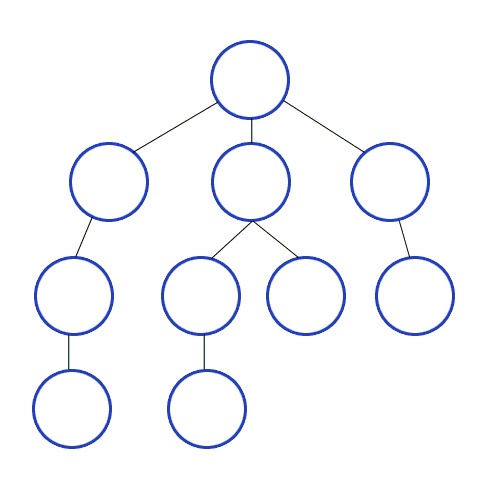
These core courses provide a comprehensive overview of the fundamental concepts in computer science.
2. Programming Fundamentals: The Building Blocks
Programming is the cornerstone of computer science. It’s the art of instructing computers to perform specific tasks by writing code in a programming language. Mastering programming fundamentals is essential for any aspiring computer scientist.
2.1. Key Concepts in Programming Fundamentals
- Variables: Named storage locations that hold data values.
- Data Types: Classifications of data, such as integers, floating-point numbers, characters, and booleans.
- Operators: Symbols that perform operations on data, such as arithmetic, comparison, and logical operations.
- Control Structures: Statements that control the flow of execution in a program, such as if-else statements, loops (for, while), and switch statements.
- Functions: Reusable blocks of code that perform specific tasks.
- Arrays: Collections of elements of the same data type, stored in contiguous memory locations.
- Object-Oriented Programming (OOP): A programming paradigm that organizes code into objects, which encapsulate data and methods (functions).
2.2. Popular Programming Languages for Beginners
- Python: Known for its readability and versatility, Python is a great choice for beginners. It’s used in web development, data science, and artificial intelligence.
- Why Python? According to a study by the University of California, Berkeley, Python’s simple syntax significantly reduces the learning curve for novice programmers.
- Java: A widely used language for enterprise applications, Android development, and more. Java is known for its platform independence.
- JavaScript: The language of the web, JavaScript is essential for front-end and back-end web development.
- C++: A powerful language used for system programming, game development, and high-performance applications. C++ provides more control over hardware resources.
2.3. Learning to Code: A Practical Approach
- Choose a Language: Select a language based on your interests and career goals.
- Start with Basics: Learn the fundamental concepts of programming, such as variables, data types, and control structures.
- Practice Regularly: Write code every day to reinforce your understanding and develop your skills.
- Work on Projects: Build small projects to apply your knowledge and gain experience.
- Seek Help: Don’t be afraid to ask for help from online forums, communities, or mentors.
- Read Code: Study code written by experienced programmers to learn new techniques and best practices.
- Contribute to Open Source: Contribute to open-source projects to gain real-world experience and collaborate with other developers.
LEARNS.EDU.VN offers a variety of programming courses and resources to help you get started on your coding journey. With a structured approach and dedicated practice, you can master the fundamentals of programming and unlock endless possibilities.
3. Data Structures and Algorithms: The Heart of Efficient Computing
Data structures and algorithms are fundamental to computer science. They provide the tools and techniques for organizing and processing data efficiently.
3.1. Understanding Data Structures
A data structure is a particular way of organizing and storing data in a computer so that it can be used efficiently. Different kinds of data structures are suited to different kinds of applications, and some are highly specialized for specific tasks.
- Arrays: A basic data structure that stores elements of the same data type in contiguous memory locations.
- Linked Lists: A linear data structure where elements are linked together using pointers.
- Stacks: A data structure that follows the Last-In-First-Out (LIFO) principle.
- Queues: A data structure that follows the First-In-First-Out (FIFO) principle.
- Trees: A hierarchical data structure where elements are organized in a parent-child relationship.
- Graphs: A non-linear data structure that consists of nodes (vertices) and edges.
- Hash Tables: A data structure that uses a hash function to map keys to values, providing fast lookups.
3.2. Key Algorithm Concepts
An algorithm is a step-by-step procedure for solving a problem or performing a task. Algorithms are essential for writing efficient and effective computer programs.
- Sorting Algorithms: Algorithms for arranging elements in a specific order, such as bubble sort, insertion sort, merge sort, and quicksort.
- Searching Algorithms: Algorithms for finding a specific element in a data structure, such as linear search and binary search.
- Graph Algorithms: Algorithms for solving problems on graphs, such as shortest path algorithms (Dijkstra’s algorithm, Bellman-Ford algorithm) and minimum spanning tree algorithms (Prim’s algorithm, Kruskal’s algorithm).
- Dynamic Programming: A technique for solving complex problems by breaking them down into smaller overlapping subproblems.
- Greedy Algorithms: A technique for making locally optimal choices at each step in the hope of finding a global optimum.
- Divide and Conquer: A technique for solving problems by recursively breaking them down into smaller subproblems until they become simple enough to solve directly.
3.3. Importance of Data Structures and Algorithms
- Efficiency: Choosing the right data structure and algorithm can significantly improve the performance of a program.
- Scalability: Data structures and algorithms enable programs to handle large amounts of data efficiently.
- Problem-Solving: Understanding data structures and algorithms is essential for solving complex problems in computer science.
- Job Interviews: Knowledge of data structures and algorithms is a key requirement for many software engineering job interviews.
3.4. Resources for Learning Data Structures and Algorithms
- Textbooks: “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein.
- Online Courses: Coursera, Udacity, edX, and Khan Academy offer courses on data structures and algorithms.
- Coding Platforms: LeetCode, HackerRank, and Codeforces provide practice problems and coding challenges to help you improve your skills.
LEARNS.EDU.VN provides in-depth courses and tutorials on data structures and algorithms, designed to equip you with the knowledge and skills you need to succeed in computer science.
4. Discrete Mathematics: The Foundation of Computer Science
Discrete mathematics is the branch of mathematics that deals with discrete objects, such as integers, graphs, and logical statements. It provides the mathematical foundations for computer science.
4.1. Key Topics in Discrete Mathematics
- Logic: The study of reasoning and argumentation, including propositional logic and predicate logic.
- Set Theory: The study of sets, which are collections of objects.
- Combinatorics: The study of counting and arranging objects.
- Graph Theory: The study of graphs, which are mathematical structures used to model relationships between objects.
- Number Theory: The study of integers and their properties.
- Proof Techniques: Methods for proving mathematical statements, such as direct proof, proof by contradiction, and proof by induction.
4.2. Relevance to Computer Science
- Algorithm Analysis: Discrete mathematics provides the tools for analyzing the correctness and efficiency of algorithms.
- Data Structures: Graph theory is used to model and analyze data structures such as trees and graphs.
- Database Theory: Set theory and logic are used to formalize database concepts and query languages.
- Cryptography: Number theory is used in cryptography to design secure encryption algorithms.
- Artificial Intelligence: Logic and probability theory are used in artificial intelligence to develop intelligent systems.
4.3. Resources for Learning Discrete Mathematics
- Textbooks: “Discrete Mathematics and Its Applications” by Kenneth H. Rosen, “Concrete Mathematics” by Ronald L. Graham, Donald E. Knuth, and Oren Patashnik.
- Online Courses: Coursera, Udacity, edX, and MIT OpenCourseWare offer courses on discrete mathematics.
- Practice Problems: Work through practice problems to reinforce your understanding and develop your problem-solving skills.
LEARNS.EDU.VN offers comprehensive courses on discrete mathematics, designed to provide you with the mathematical foundations you need to succeed in computer science.
5. Computer Architecture: Understanding the Hardware
Computer architecture is the study of the design and organization of computer systems. It focuses on how hardware components interact to execute instructions and process data.
5.1. Key Components of a Computer System
- Central Processing Unit (CPU): The brain of the computer, responsible for executing instructions.
- Memory: Stores data and instructions that the CPU needs to access quickly.
- Input/Output (I/O) Devices: Allow the computer to interact with the outside world, such as keyboards, mice, monitors, and storage devices.
- Buses: Communication pathways that connect the various components of the computer system.
5.2. Topics Covered in Computer Architecture
- Instruction Set Architecture (ISA): The interface between hardware and software, defining the instructions that the CPU can execute.
- CPU Design: The design of the CPU, including the control unit, arithmetic logic unit (ALU), and registers.
- Memory Hierarchy: The organization of memory into different levels, such as cache, main memory, and secondary storage.
- Pipelining: A technique for improving CPU performance by overlapping the execution of multiple instructions.
- Parallel Processing: The use of multiple CPUs to execute instructions simultaneously.
- I/O Systems: The design of I/O devices and interfaces, including DMA (Direct Memory Access).
5.3. Relevance to Computer Science
- Performance Optimization: Understanding computer architecture allows you to optimize software for better performance.
- System Programming: Knowledge of computer architecture is essential for writing system-level software, such as operating systems and device drivers.
- Embedded Systems: Computer architecture is critical for designing embedded systems, which are specialized computer systems designed for specific tasks.
5.4. Resources for Learning Computer Architecture
- Textbooks: “Computer Organization and Design” by David A. Patterson and John L. Hennessy, “Structured Computer Organization” by Andrew S. Tanenbaum and Todd Austin.
- Online Courses: Coursera, Udacity, edX, and MIT OpenCourseWare offer courses on computer architecture.
- Simulation Tools: Use simulation tools such as Logisim and Simulators to design and simulate computer systems.
LEARNS.EDU.VN offers detailed courses on computer architecture, providing you with a solid understanding of how computer systems work at the hardware level.
6. Operating Systems: Managing Computer Resources
An operating system (OS) is a software that manages computer hardware and provides services for applications. It acts as an intermediary between the hardware and the applications, providing a platform for them to run.
6.1. Key Functions of an Operating System
- Process Management: Managing the execution of processes, including creating, scheduling, and terminating processes.
- Memory Management: Allocating and managing memory resources for processes.
- File System Management: Organizing and managing files and directories on storage devices.
- Input/Output (I/O) Management: Handling communication between the computer and I/O devices.
- Security: Protecting the computer system from unauthorized access and malicious software.
- User Interface: Providing a user interface for interacting with the computer system.
6.2. Topics Covered in Operating Systems
- Process Scheduling: Algorithms for scheduling processes, such as First-Come-First-Served (FCFS), Shortest Job First (SJF), and Round Robin.
- Memory Management: Techniques for managing memory, such as paging, segmentation, and virtual memory.
- File Systems: Design and implementation of file systems, including file allocation methods and directory structures.
- Concurrency: Techniques for managing concurrent access to shared resources, such as locks, semaphores, and monitors.
- Deadlocks: Conditions where processes are blocked indefinitely, waiting for each other to release resources.
- Security: Mechanisms for protecting the computer system from security threats, such as authentication, authorization, and access control.
6.3. Relevance to Computer Science
- System Programming: Understanding operating systems is essential for writing system-level software, such as device drivers and system utilities.
- Application Development: Operating systems provide the platform for running applications, so understanding how they work can help you write better applications.
- Cloud Computing: Operating systems are a key component of cloud computing infrastructure.
6.4. Resources for Learning Operating Systems
- Textbooks: “Operating System Concepts” by Abraham Silberschatz, Peter Baer Galvin, and Greg Gagne, “Modern Operating Systems” by Andrew S. Tanenbaum.
- Online Courses: Coursera, Udacity, edX, and MIT OpenCourseWare offer courses on operating systems.
- Operating System Projects: Implement your own operating system or contribute to an existing open-source operating system.
LEARNS.EDU.VN offers in-depth courses on operating systems, providing you with the knowledge and skills you need to design, implement, and manage computer systems effectively.
7. Database Management Systems: Organizing and Managing Data
A database management system (DBMS) is a software that allows you to store, retrieve, and manage large amounts of structured data efficiently.
7.1. Key Concepts in Database Management Systems
- Data Models: Conceptual models for representing data, such as the relational model, the entity-relationship model, and the object-oriented model.
- Database Design: The process of designing the structure of a database, including tables, columns, and relationships.
- SQL (Structured Query Language): A standard language for querying and manipulating data in relational databases.
- Transactions: Sequences of operations that are treated as a single unit of work, ensuring data consistency and integrity.
- Concurrency Control: Mechanisms for managing concurrent access to the database, ensuring that transactions do not interfere with each other.
- Data Integrity: Ensuring the accuracy and consistency of data in the database.
- Database Security: Protecting the database from unauthorized access and malicious activities.
7.2. Types of Database Management Systems
- Relational Database Management Systems (RDBMS): Store data in tables with rows and columns, and use SQL for querying and manipulation. Examples include MySQL, PostgreSQL, Oracle, and Microsoft SQL Server.
- NoSQL Database Management Systems: Store data in a variety of formats, such as key-value pairs, documents, and graphs. Examples include MongoDB, Cassandra, and Redis.
7.3. Relevance to Computer Science
- Data-Driven Applications: Databases are essential for building data-driven applications, such as e-commerce websites, social media platforms, and business intelligence systems.
- Data Analysis: Databases are used for storing and analyzing large amounts of data, enabling organizations to make better decisions.
- Web Development: Databases are used to store and manage data for web applications.
7.4. Resources for Learning Database Management Systems
- Textbooks: “Database System Concepts” by Abraham Silberschatz, Henry F. Korth, and S. Sudarshan, “Fundamentals of Database Systems” by Ramez Elmasri and Shamkant B. Navathe.
- Online Courses: Coursera, Udacity, edX, and Khan Academy offer courses on database management systems.
- Database Projects: Design and implement your own database for a specific application.
LEARNS.EDU.VN offers comprehensive courses on database management systems, providing you with the knowledge and skills you need to design, implement, and manage databases effectively.
8. Computer Networks: Connecting the World
A computer network is a collection of interconnected computers and devices that can communicate and share resources.
8.1. Key Concepts in Computer Networks
- Network Protocols: Standards that govern communication between devices on a network, such as TCP/IP, HTTP, and DNS.
- Network Topologies: The physical or logical arrangement of devices on a network, such as bus, star, ring, and mesh.
- Network Layers: The division of network functionality into layers, such as the OSI model and the TCP/IP model.
- IP Addressing: A system for assigning unique addresses to devices on a network.
- Routing: The process of forwarding data packets between networks.
- Network Security: Protecting the network from unauthorized access and malicious activities.
8.2. Types of Computer Networks
- Local Area Network (LAN): A network that connects devices in a limited area, such as a home, office, or school.
- Wide Area Network (WAN): A network that connects devices over a large geographical area, such as the internet.
- Wireless Local Area Network (WLAN): A network that uses wireless technology to connect devices, such as Wi-Fi.
8.3. Relevance to Computer Science
- Internet: Computer networks are the foundation of the internet, enabling communication and data sharing across the world.
- Cloud Computing: Computer networks are essential for cloud computing, providing the infrastructure for delivering services over the internet.
- Web Development: Web applications rely on computer networks to communicate between clients and servers.
8.4. Resources for Learning Computer Networks
- Textbooks: “Computer Networking: A Top-Down Approach” by James F. Kurose and Keith W. Ross, “Computer Networks” by Andrew S. Tanenbaum and David J. Wetherall.
- Online Courses: Coursera, Udacity, edX, and Khan Academy offer courses on computer networks.
- Network Simulation Tools: Use network simulation tools such as Wireshark and GNS3 to analyze and simulate network traffic.
LEARNS.EDU.VN provides comprehensive courses on computer networks, giving you the knowledge and skills you need to design, implement, and manage computer networks effectively.
9. Software Engineering: Building Reliable Software
Software engineering is the discipline of designing, developing, and maintaining large software systems.
9.1. Key Principles of Software Engineering
- Requirements Analysis: Understanding and documenting the requirements of the software system.
- Design: Creating a blueprint for the software system, including the architecture, modules, and interfaces.
- Coding: Writing the source code for the software system.
- Testing: Verifying that the software system meets the requirements and works correctly.
- Maintenance: Fixing bugs, adding new features, and improving the performance of the software system.
- Project Management: Planning, organizing, and managing the development of the software system.
9.2. Software Development Methodologies
- Waterfall Model: A linear, sequential approach to software development, where each phase is completed before moving on to the next.
- Agile Development: An iterative, incremental approach to software development, where the requirements and solutions evolve through collaboration between self-organizing, cross-functional teams.
- Scrum: A specific agile framework for managing software development projects.
9.3. Best Practices in Software Engineering
- Code Reviews: Having other developers review your code to identify bugs and improve code quality.
- Version Control: Using a version control system, such as Git, to track changes to the source code.
- Continuous Integration: Automating the process of building, testing, and deploying the software system.
- Automated Testing: Writing automated tests to verify that the software system works correctly.
- Design Patterns: Using proven solutions to common design problems.
9.4. Relevance to Computer Science
- Large-Scale Systems: Software engineering principles are essential for building large-scale software systems that are reliable, scalable, and maintainable.
- Teamwork: Software engineering emphasizes teamwork and collaboration.
- Career Opportunities: Software engineering is a high-demand field with numerous career opportunities.
9.5. Resources for Learning Software Engineering
- Textbooks: “Software Engineering” by Ian Sommerville, “Clean Code” by Robert C. Martin.
- Online Courses: Coursera, Udacity, edX, and MIT OpenCourseWare offer courses on software engineering.
- Software Projects: Work on real-world software projects to gain experience and apply software engineering principles.
LEARNS.EDU.VN offers comprehensive courses on software engineering, providing you with the knowledge and skills you need to build reliable and scalable software systems.
10. Specialized Areas in Computer Science
Beyond the core subjects, computer science offers a wide range of specialized areas that you can explore:
10.1. Artificial Intelligence (AI)
AI is the field of computer science that deals with the design and development of intelligent systems that can perform tasks that typically require human intelligence, such as learning, reasoning, and problem-solving.
- Machine Learning: A subset of AI that focuses on developing algorithms that can learn from data without being explicitly programmed.
- Natural Language Processing (NLP): A field of AI that deals with the interaction between computers and human languages.
- Computer Vision: A field of AI that deals with enabling computers to see and interpret images.
- Robotics: A field of AI that deals with the design, construction, operation, and application of robots.
10.2. Cybersecurity
Cybersecurity is the practice of protecting computer systems, networks, and data from unauthorized access, theft, and damage.
- Network Security: Protecting computer networks from security threats.
- Cryptography: The art of encrypting and decrypting data to protect it from unauthorized access.
- Ethical Hacking: Testing the security of computer systems and networks by simulating attacks.
- Incident Response: Responding to and recovering from security incidents.
10.3. Web Development
Web development is the process of creating websites and web applications.
- Front-End Development: Developing the user interface of websites and web applications using HTML, CSS, and JavaScript.
- Back-End Development: Developing the server-side logic and databases for websites and web applications using languages such as Python, Java, and PHP.
- Full-Stack Development: Developing both the front-end and back-end of websites and web applications.
10.4. Mobile App Development
Mobile app development is the process of creating applications for mobile devices, such as smartphones and tablets.
- iOS Development: Developing applications for Apple’s iOS platform using Swift or Objective-C.
- Android Development: Developing applications for Google’s Android platform using Java or Kotlin.
- Cross-Platform Development: Developing applications that can run on multiple platforms using frameworks such as React Native and Flutter.
10.5. Game Development
Game development is the process of creating video games.
- Game Design: Designing the gameplay, story, and characters of a video game.
- Game Programming: Writing the code for the video game using languages such as C++ and C#.
- Game Art: Creating the visual assets for a video game, such as characters, environments, and user interfaces.
10.6. Data Science
Data science is an interdisciplinary field that uses scientific methods, processes, algorithms, and systems to extract knowledge and insights from structured and unstructured data.
- Data Analysis: The process of inspecting, cleaning, transforming, and modeling data to discover useful information, draw conclusions, and support decision-making.
- Data Visualization: The graphical representation of data to make it easier to understand and interpret.
- Big Data: The processing and analysis of large and complex datasets.
LEARNS.EDU.VN offers courses and resources in each of these specialized areas, helping you explore your interests and develop the skills you need to succeed in your chosen field.
11. Real-World Applications of Computer Science
Computer science is not just an academic discipline; it has numerous real-world applications that impact our lives every day.
11.1. Healthcare
- Medical Imaging: Computer algorithms are used to analyze medical images, such as X-rays and MRIs, to detect diseases and abnormalities.
- Electronic Health Records (EHRs): Databases are used to store and manage patient medical records, improving efficiency and coordination of care.
- Telemedicine: Computer networks are used to provide remote healthcare services, such as virtual consultations and remote monitoring.
- Drug Discovery: Computer simulations are used to model the interactions between drugs and biological molecules, accelerating the drug discovery process.
11.2. Finance
- Algorithmic Trading: Computer algorithms are used to execute trades automatically, based on predefined rules and market conditions.
- Fraud Detection: Machine learning algorithms are used to detect fraudulent transactions and prevent financial losses.
- Risk Management: Computer models are used to assess and manage financial risks.
11.3. Transportation
- Autonomous Vehicles: Computer vision, machine learning, and robotics are used to develop self-driving cars and trucks.
- Traffic Management: Computer algorithms are used to optimize traffic flow and reduce congestion.
- Logistics: Computer systems are used to manage supply chains and optimize delivery routes.
11.4. Education
- Online Learning: Computer networks and web technologies are used to deliver online courses and educational resources.
- Personalized Learning: Machine learning algorithms are used to personalize the learning experience for each student, based on their individual needs and preferences.
- Educational Games: Video games are used to engage students and make learning more fun and interactive.
11.5. Entertainment
- Video Games: Computer science is the foundation of the video game industry, from game design and programming to game art and animation.
- Streaming Services: Computer networks and web technologies are used to deliver streaming video and music services.
- Special Effects: Computer graphics and animation are used to create special effects in movies and television shows.
These are just a few examples of the many real-world applications of computer science. As technology continues to evolve, computer science will play an increasingly important role in shaping our world.
12. Essential Skills for a Computer Science Career
In addition to technical knowledge, a successful computer science career requires a set of essential skills.
12.1. Problem-Solving Skills
The ability to analyze complex problems and develop logical solutions is crucial for any computer science professional.
- Break Down Problems: Decompose complex problems into smaller, manageable parts.
- Identify Patterns: Recognize patterns and relationships in data.
- Develop Algorithms: Design step-by-step procedures for solving problems.
- Test Solutions: Verify that your solutions work correctly and efficiently.
12.2. Analytical Thinking
The ability to evaluate information, identify trends, and draw conclusions based on evidence is essential for making informed decisions.
- Critical Thinking: Evaluate information objectively and identify biases.
- Data Analysis: Analyze data to identify trends and patterns.
- Statistical Reasoning: Apply statistical methods to analyze data and draw conclusions.
12.3. Communication Skills
The ability to communicate effectively with colleagues, clients, and stakeholders is crucial for collaborating on projects and conveying technical information.
- Verbal Communication: Clearly and concisely explain technical concepts to non-technical audiences.
- Written Communication: Write clear and concise documentation, reports, and emails.
- Presentation Skills: Deliver engaging and informative presentations.
- Active Listening: Pay attention to what others are saying and ask clarifying questions.
12.4. Teamwork and Collaboration
The ability to work effectively in a team and collaborate with others is essential for building large software systems.
- Collaboration Tools: Use collaboration tools such as Git, Slack, and Jira to communicate and coordinate with team members.
- Conflict Resolution: Resolve conflicts constructively and find common ground.
- Empathy: Understand and respect the perspectives of others.
12.5. Continuous Learning
The field of computer science is constantly evolving, so the ability to learn new technologies and adapt to change is crucial for staying relevant and competitive.
- Stay Updated: Follow industry news, read blogs, and attend conferences to stay updated on the latest trends and technologies.
- Online Courses: Take online courses to learn new skills and expand your knowledge.
- Personal Projects: Work on personal projects to experiment with new technologies and build your portfolio.
LEARNS.EDU.VN provides resources and support to help you develop these essential skills, preparing you for a successful and rewarding computer science career.
13. Career Paths in Computer Science
A computer science degree can open doors to a wide range of exciting and rewarding career paths:
- Software Engineer: Develops software applications for various platforms and industries.
- Web Developer: Creates websites and web applications.
- Data Scientist: Analyzes data to extract insights and support decision-making.
- Database Administrator: Manages and maintains databases.
- Network Administrator: Manages and maintains computer networks.
- Cybersecurity Analyst: Protects computer systems and networks from security threats.
- Artificial Intelligence Engineer: Develops AI systems and algorithms.
- Game Developer: Creates video games.
- Mobile App Developer: Develops applications for mobile devices.
- Computer Systems Analyst: Analyzes an organization’s computer systems and recommends improvements.
- IT Project Manager: Plans, organizes, and manages IT projects.
- Computer and Information Research Scientist: Conducts research in computer science and develops new technologies.
The demand for computer science professionals is high and growing, with excellent job prospects and competitive salaries. According to the U.S. Bureau of Labor Statistics, the median annual wage for computer and information technology occupations was $97,430 in May 2022.
14. Resources for Learning Computer Science
There are numerous resources available to help you learn computer science, both online and offline:
- Online Courses: Coursera, Udacity, edX, and Khan Academy offer a wide range of computer science courses, from introductory to advanced levels.
- Textbooks: Many excellent textbooks cover various topics in computer science, providing a comprehensive and in-depth understanding of the subject.
- Coding Platforms: LeetCode, HackerRank, and Codeforces provide practice problems and coding challenges to help you improve your skills.
- Open Source Projects: Contributing to open-source projects is a great way to gain real-world experience and collaborate with other developers.
- Online Communities: Online forums and communities, such as Stack Overflow and Reddit, provide a platform for asking questions, sharing knowledge, and connecting with other computer science enthusiasts.
- Universities and Colleges: Many universities and colleges offer computer science degree programs, providing a structured and comprehensive education in the field.
learns.edu.vn offers a wealth of resources for learning computer science, including courses, tutorials, articles, and community forums. Whether you’re a beginner or an experienced professional, you’ll find something to help you expand your knowledge